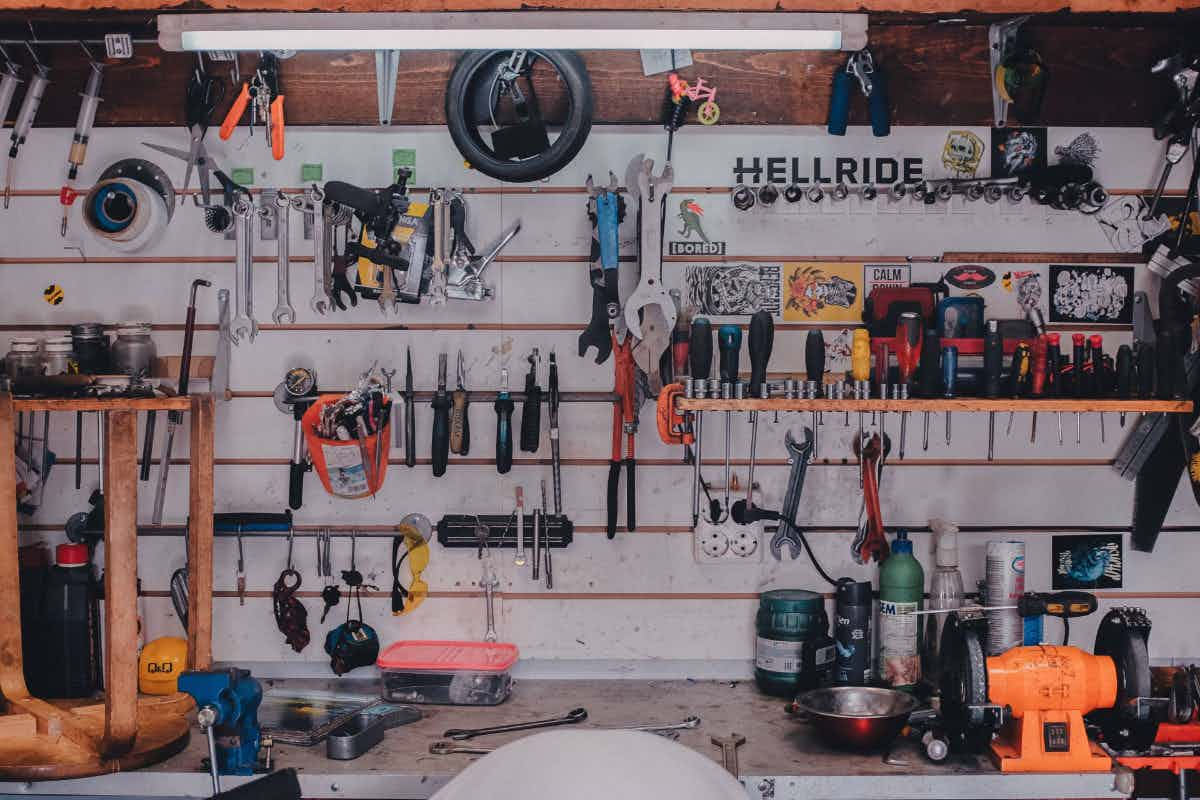
In this post, we'll explore how to create Ruby modules that are configurable by users of our code — a pattern that allows gem authors to add more flexibility to their libraries.
Most Ruby developers are familiar with using modules to share behavior. After all, this is one of their main use cases, according to the documentation:
Modules serve two purposes in Ruby, namespacing and mixin functionality.
Rails added some syntactic sugar in the form of ActiveSupport::Concern
, but the general principle remains the same.
The Problem
Using modules to provide mixin functionality is usually straightforward. All we have to do is bundle up some methods and include our module elsewhere:
module HelloWorld def hello "Hello, world!" end end class Test include HelloWorld end Test.new.hello #=> "Hello, world!"
This is a pretty static mechanism, though Ruby's inherited
and extended
hook methods allow for some varying behavior based on the including class:
module HelloWorld def self.included(base) define_method :hello do "Hello, world from #{base}!" end end end class Test include HelloWorld end Test.new.hello #=> "Hello, world from Test!"
This is somewhat more dynamic but still doesn't allow our code users to, for instance, rename the hello
method at module inclusion time.
The Solution: Configurable Ruby Modules
Over the past few years, a new pattern has emerged that solves this problem, which people sometimes refer to as the "module builder pattern". This technique relies on two primary features of Ruby:
-
Modules are just like any other objects—they can be created on the fly, assigned to variables, dynamically modified, as well as passed to or returned from methods.
rubydef make_module # create a module on the fly and assign it to variable mod = Module.new # modify module mod.module_eval do def hello "Hello, AppSignal world!" end end # explicitly return it mod end
-
The argument to
include
orextend
calls doesn't have to be a module, it can also be an expression returning one, e.g. a method call.rubyclass Test # include the module returned by make_module include make_module end Test.new.hello #=> "Hello, AppSignal world!"
Module Builder in Action
We will now use this knowledge to build a simple module called Wrapper
, which implements the following behavior:
- A class including
Wrapper
can only wrap objects of a specific type. The constructor will verify the argument type and raise an error if the type doesn't match what's expected. - The wrapped object will be available through an instance method called
original_<class>
, e.g.original_integer
ororiginal_string
. - It will allow consumers of our code to specify an alternative name for this accessor method, for example,
the_string
.
Let's take a look at how we want our code to behave:
# 1 class IntWrapper # 2 include Wrapper.for(Integer) end # 3 i = IntWrapper.new(42) i.original_integer #=> 42 # 4 i = IntWrapper.new("42") #=> TypeError (not a Integer) # 5 class StringWrapper include Wrapper.for(String, accessor_name: :the_string) end s = StringWrapper.new("Hello, World!") # 6 s.the_string #=> "Hello, World!"
In step 1, we define a new class called IntWrapper
.
In step 2, we ensure that this class doesn't simply include a module by name but instead, mixes in the result of a call to Wrapper.for(Integer)
.
In step 3, we instantiate an object of our new class and assign it to i
. As specified, this object has a method called original_integer
, that satisfies one of our requirements.
In step 4, if we try to pass in an argument of the wrong type, like a string, a helpful TypeError
will be raised. Finally, let's verify that users are able to specify custom accessor names.
For this, we define a new class called StringWrapper
in step 5 and pass the_string
as the keyword argument accessor_name
, which we see in action in step 6.
While this is admittedly a somewhat contrived example, it has just enough varying behavior to show off the module builder pattern and how it is used.
First Attempt
Based on the requirements and usage example, we can now begin the implementation. We already know that we need a module named Wrapper
with a module-level method called for
, which takes a class as an optional keyword argument:
module Wrapper def self.for(klass, accessor_name: nil) end end
Since the return value of this method becomes the argument to include
, it needs to be a module. Thus, we can create a new anonymous one with Module.new
.
Module.new do end
As per our requirements, this needs to define a constructor which verifies the type of the passed-in object, as well as an appropriately named accessor method. Let's start with the constructor:
define_method :initialize do |object| raise TypeError, "not a #{klass}" unless object.is_a?(klass) @object = object end
This piece of code uses define_method
to dynamically add an instance method to the receiver. Since the block acts as a closure, it can use the klass
object from the outer scope to perform the required type check.
Adding an appropriately named accessor method is not that much harder:
# 1 method_name = accessor_name || begin klass_name = klass.to_s.gsub(/(.)([A-Z])/,'\1_\2').downcase "original_#{klass_name}" end # 2 define_method(method_name) { @object }
First, we need to see if the caller of our code passed in an accessor_name
. If so, we just assign it to method_name
and are then done. Otherwise, we take the class and convert it to an underscored string, for instance, Integer
turns into integer
or OpenStruct
into open_struct
. This klass_name
variable is then prefixed with original_
to generate the final accessor name. Once we know the method’s name, we again use define_method
to add it to our module, as shown in step 2.
Here's the complete code up to this point. Less than 20 lines for a flexible and configurable Ruby module; not too bad.
module Wrapper def self.for(klass, accessor_name: nil) Module.new do define_method :initialize do |object| raise TypeError, "not a #{klass}" unless object.is_a?(klass) @object = object end method_name = accessor_name || begin klass_name = klass.to_s.gsub(/(.)([A-Z])/,'\1_\2').downcase "original_#{klass_name}" end define_method(method_name) { @object } end end end
Observant readers might remember that Wrapper.for
returns an anonymous module. This isn't a problem, but can get a bit confusing when examining an object's inheritance chain:
StringWrapper.ancestors #=> [StringWrapper, #<Module:0x0000000107283680>, Object, Kernel, BasicObject]
Here #<Module:0x0000000107283680>
(the name will vary if you are following along) refers to our anonymous module.
Improved Version
Let's make life easier for our users by returning a named module instead of an anonymous one. The code for this is very similar to what we had before, with some minor changes:
module Wrapper def self.for(klass, accessor_name: nil) # 1 mod = const_set("#{klass}InstanceMethods", Module.new) # 2 mod.module_eval do define_method :initialize do |object| raise TypeError, "not a #{klass}" unless object.is_a?(klass) @object = object end method_name = accessor_name || begin klass_name = klass.to_s.gsub(/(.)([A-Z])/, '\1_\2').downcase "original_#{klass_name}" end define_method(method_name) { @object } end # 3 mod end end
In the first step, we create a nested module called "#{klass}InstanceMethods"
(for example IntegerInstanceMethods
), that is just an “empty” module.
As shown in step 2, we use module_eval
in the for
method, which evaluates a block of code in the context of the module it’s called on. This way, we can add behavior to the module before returning it in step 3.
If we now examine the ancestors of a class including Wrapper
, the output will include a properly named module, which is much more meaningful and easier to debug than the previous anonymous module.
StringWrapper.ancestors #=> [StringWrapper, Wrapper::StringInstanceMethods, Object, Kernel, BasicObject]
The Module Builder Pattern in the Wild
Apart from this post, where else can we find the module builder pattern or similar techniques?
One example is the dry-rb
family of gems, where, for example, dry-effects
uses module builders to pass configuration options to the various effect handlers:
# This adds a `counter` effect provider. It will handle (eliminate) effects include Dry::Effects::Handler.State(:counter) # Providing scope is required # All cache values will be scoped with this key include Dry::Effects::Handler.Cache(:blog)
We can find similar usage in the excellent Shrine gem, which provides a file upload toolkit for Ruby applications:
class Photo < Sequel::Model include Shrine::Attachment(:image) end
This pattern is still relatively new, but I expect we'll see more of it in the future, especially in gems that focus more on pure Ruby applications than Rails ones.
Summary
In this post, we explored how to implement configurable modules in Ruby, a technique sometimes referred to as the module builder pattern. Like other metaprogramming techniques, this comes at the cost of increased complexity and therefore shouldn't be used without good reason. However, in the rare cases where such flexibility is needed, Ruby's object model once again allows for an elegant and concise solution. The module builder pattern isn't something most Ruby developers will need often, but it's a great tool to have in one's toolkit, especially for library authors.
P.S. If you'd like to read Ruby Magic posts as soon as they get off the press, subscribe to our Ruby Magic newsletter and never miss a single post!