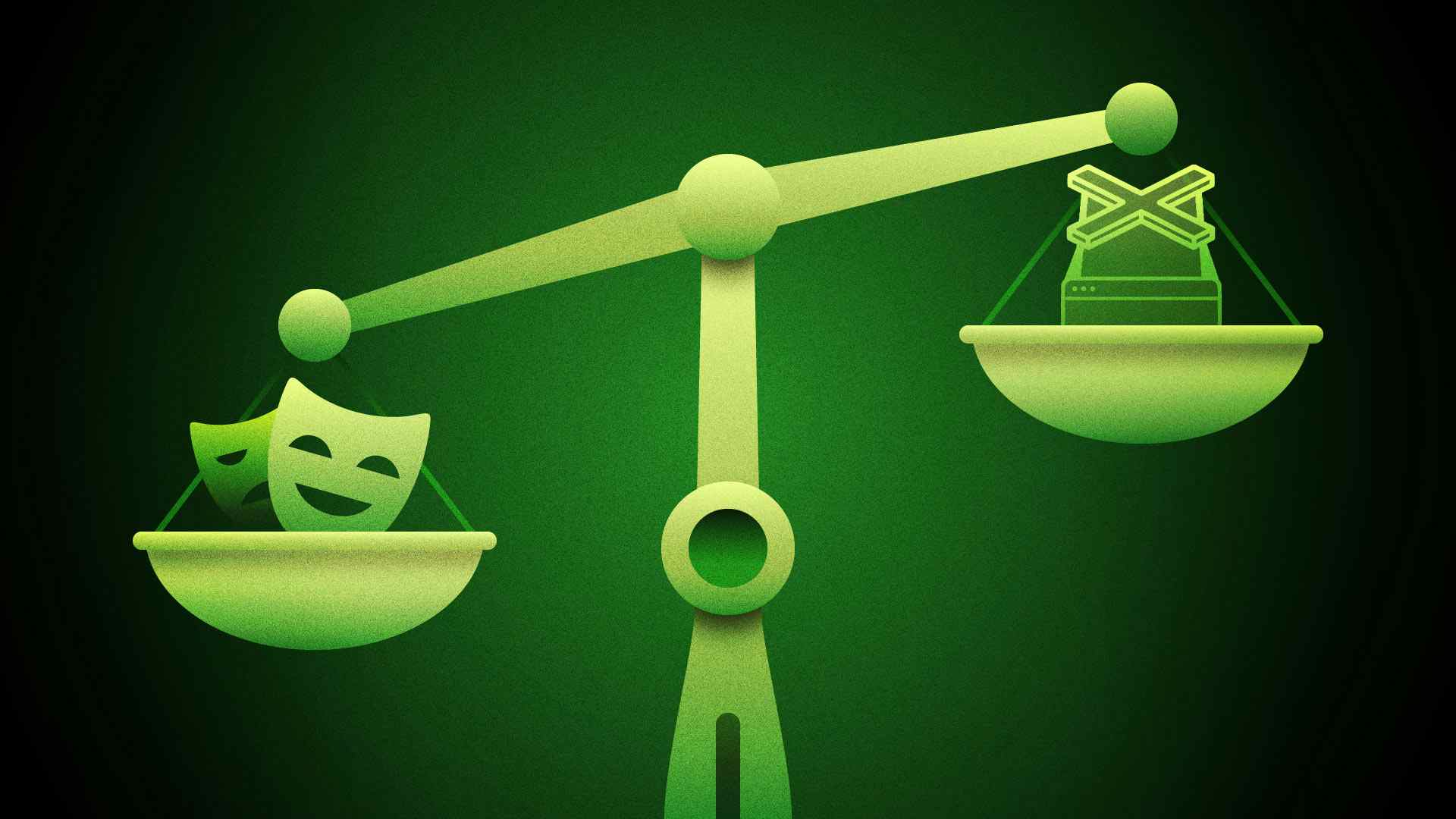
Playwright and Puppeteer have emerged as two of the most powerful end-to-end testing Node.js libraries. Their robust APIs make it easier to test the UI and workflows of sites and web applications.
Although they are similar tools that offer comparable features, there are circumstances in which one is better to use than the other.
In this article, we'll look at scenarios where it's better to use Playwright than Puppeteer for functionality and performance reasons. By understanding which tool is best for your specific use case, you can build a quality-oriented, efficient, and reliable testing process.
It's time to become a Playwright expert!
Playwright and Puppeteer for Node.js: Getting Started
Playwright and Puppeteer are powerful tools for automating browsers and testing web applications.
Playwright is an open-source Node.js library for end-to-end testing developed by Microsoft and launched in 2020. It's characterized by its powerful cross-browser API that controls Chrome, Firefox, and Safari. This means you can write your testing automation scripts once and run them across multiple browsers seamlessly. Playwright's API is designed to be easy to use, with concise and readable syntax.
Similarly, Puppeteer is also an open-source Node.js library for automation testing, originally released in 2017 by Google's development team. It provides a high-level API for controlling headless Chrome or Chromium-based browsers. Puppeteer is widely used for automating user interactions on web pages, taking screenshots, and scraping data. It offers a rich set of features and fine-grained control over browser behavior.
The two libraries have comparable goals and a similar nature. They both excel in automating web browser tasks, but there are some notable differences between them. Playwright is preferable to Puppeteer in specific scenarios.
Let's find out when and why!
5 Reasons to Use Playwright Over Puppeteer for Node.js
Here are some of the most popular reasons why you should go for Playwright instead of Puppeteer for browser automation.
1. Ease of Use
Playwright development began three years after the first release of Puppeteer. In the IT world, that's a huge time advantage! Playwright had the opportunity to learn from the challenges and setbacks that shaped Puppeteer's development.
So, Playwright's API was designed to be intuitive and developer-friendly since day one. Users of all experience levels can use it, as the developers behind it have put significant effort into ensuring that it's straightforward and consistent. This makes Playwright easier to learn and adopt than Puppeteer.
As part of this effort, Playwright prioritizes its community. The team is always looking for feedback and ways to address users' needs. This commitment to community support has led to a vibrant ecosystem, which includes active forums, open-source contributions, and a Discord server.
Also, Playwright's documentation is up-to-date, comprehensive, and well-structured. It provides clear explanations and useful examples to guide developers through the browser automation journey. Plus, its site offers tutorials, guides, and a complete API reference. All these elements contribute to its ease of use.
2. Cross-Browser Testing
Playwright natively offers cross-browser support, while Puppeteer works only with Chromium-based browsers. Technically, it also offers Firefox support, but that feature has been in the experimental stage for years now.
In particular, Playwright allows you to automate:
- Chromium-based browsers: Chrome, Edge, Opera
- Firefox
- WebKit-based browsers: Safari
As you can see, Playwright gives you the ability to deal with many browser engines through the same API. When it comes to cross-browser testing, that's a game-changer!
You can write test scripts once and execute them across all major browsers. Whether you're handling complex interactions, capturing screenshots, or scraping data, you don't have to adapt your logic to the target browser. Playwright's consistent and comprehensive API will do that behind the scenes for you.
In other terms, a single script enables you to verify the functionality and visual consistency of a web application across different platforms and environments. This saves you significant time and effort. Instead of having to adapt tests to browsers or rely on separate tools for each browser, you only need a single tool.
It's also important to note that Playwright keeps up with the latest browser updates. The development team works hard to ensure compatibility with the latest browser versions and features, with multiple releases a week. That guarantees that its cross-browser features remain up-to-date and in line with the evolving web landscape.
3. Multi-Language Support
Playwright and Puppeteer both offer cross-platform testing capabilities on Windows, Linux, and macOS. However, Playwright offers more flexibility than Puppeteer in terms of supported programming languages.
Even though Playwright is a Node.js library, there are official bindings for Python, .NET, and Java. This expands its reach to a broader range of developers. The functions offered by Playwright's API are consistent across all these programming languages. The syntax remains almost the same, so it isn't difficult to migrate from one language to another.
The multilingual nature of Playwright makes it a versatile browser automation tool that fits into different environments. Each team can write automation scripts in the language they're more comfortable with. This leads to a better developer experience, enhancing productivity and code maintainability.
In contrast, Puppeteer is only available for Node.js. Actually, there are some community-driven ports, but they're all unofficial. Right now, Google is only focusing its efforts on Node.js.
Another difference is that Playwright is developed in TypeScript, while Puppeteer is in JavaScript. At the same time, both tools can be used in TypeScript and JavaScript with no extra dependencies required.
4. Performance
When it comes to performance, both Playwright and Puppeteer are efficient solutions. When directly comparing a script with the same logic, Puppeteer is usually slightly faster. This is because it benefits from Chrome-specific optimizations, resulting in faster execution times. After all, one of the main goals of the Puppeteer project is to have almost zero performance overhead over automated pages.
Yet Playwright offers better performance overall because of its native support for parallelism. By default, it executes test files in parallel. When given a test, Playwright starts several worker processes simultaneously behind the scenes. Each has an identical environment and launches its own browser. On the contrary, single tests within a file are executed sequentially within the same worker process. However, you can configure Playwright to run these in parallel as well.
This parallelism feature is particularly useful when dealing with large test suites. The ability to distribute test execution across numerous workers speeds up the testing process, maximizing resource usage and reducing overall execution time.
Note that Puppeteer doesn't support parallel execution for its tests. You can still achieve it, but this involves custom logic and additional dependencies.
5. Overall Features
Playwright offers a wider set of features for browser automation and testing compared to Puppeteer. These include auto-waiting, code generation, and built-in error handling capabilities.
Auto-waiting
Before taking specific actions, Playwright automatically waits for an element to be:
- Attached to the DOM
- Visible
- Stable and not in an animation
- Able to receive events
- Enabled
This feature reduces your chances of getting flaky results, improving the overall stability of your tests without the need for explicit waits in the code.
Let's compare two code snippets to highlight the benefits of automatic waits.
First, we'll look at a Puppeteer example:
const puppeteer = require("puppeteer")(async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://your-web-application.com"); // Explicitly wait for the login button to be visible await page.waitForSelector(".login-btn", { visible: true, }); await page.click(".login-btn"); // ... await browser.close(); })();
Note that you must use waitForSelector()
to wait for the button element to become visible before clicking it.
Now, let's take a look at the equivalent Playwright code:
import { test } from "@playwright/test"; test("click login button", async ({ page }) => { await page.goto(" https://your-web-application.com "); // automatic wait performed behind the scene await page.click(".login-btn"); // ... });
Playwright automatically waits for the button element to be visible and clickable before performing the click()
action.
This approach saves time, simplifies the code, and improves readability.
Code Generation
Playwright comes with a test generator that allows you to perform operations on a browser visually.
Behind the scenes, the tool records your actions and translates them into optimized tests through code generation.
Built-in Error Handling
Playwright offers a Visual Studio Code extension to live debug a test in the browser and a special debug mode that launches a built-in debugger.
All these features greatly improve the development experience Playwright offers to developers.
Playwright Vs. Puppeteer: Comparison
Let's take a look at a summary comparison table:
Tool | Playwright | Puppeteer |
---|---|---|
Developed by | Microsoft | |
Launch date | 2020 | 2017 |
Developed in | TypeScript | JavaScript |
Browsers | - Chromium-based browsers (Chrome, Edge, Opera) - Firefox - WebKit-based browser (Safari) | - Chromium-based browsers (Chrome, Edge, Opera) - Experimental support for Firefox |
Languages | JavaScript, TypeScript, Python, .NET, C#, and Java | JavaScript, TypeScript |
OSs supported | Windows, Linux, macOS | Windows, Linux, macOS |
Support for parallel test execution | ✅ | ❌ |
Features | Testing, browser automation, debugging, code generation | Testing, browser automation |
As you can see, Playwright is better in almost all the aspects we've considered.
To learn more about how to move from Puppeteer to Playwright, check out the official migration guide.
Wrapping Up: Playwright, the Cross-Platform Testing Tool For You!
In this post, we took a look at some of the most important ways in which Playwright is better than Puppeteer.
You now know:
- What Playwright and Puppeteer do, and why they are often compared
- What languages and browsers the two tools support
- What features they offer, and how to get the most out of those features
If you'd like to learn more, check out some of our other posts about Puppeteer and Playwright:
- An Introduction to Playwright for Node.js
- Pitfalls to Avoid in Playwright for Node.js
- Puppeteer in Node.js: Common Mistakes to Avoid
- Puppeteer in Node.js: More Antipatterns to Avoid
Thanks for reading!
P.S. If you liked this post, subscribe to our JavaScript Sorcery list for a monthly deep dive into more magical JavaScript tips and tricks.
P.P.S. If you need an APM for your Node.js app, go and check out the AppSignal APM for Node.js.