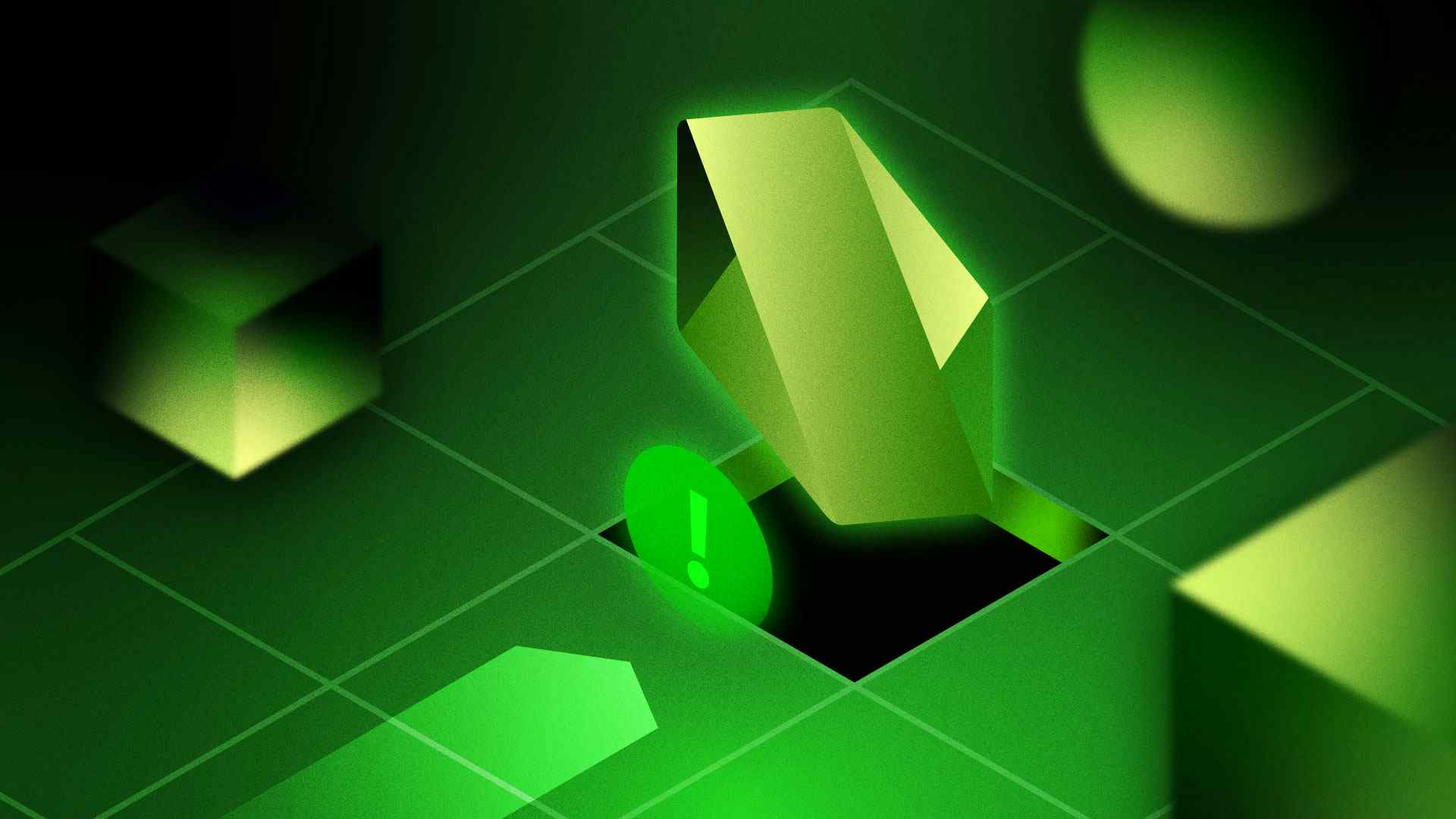
Despite its many advantages, Node.js comes with a set of potential pitfalls if you don't maintain your application properly, such as:
- outdated dependencies
- memory leaks
- improper error handling
- overcomplicated code
- neglecting security practices
- blocking code
- callback hell
- the overuse of global variables
In this post, we'll explore these pitfalls and how to avoid them.
Let's dive straight in!
Outdated Dependencies
Keep your dependencies up-to-date to avoid potential security issues and ensure your applications run on the most up-to-date version of Node.js.
Outdated dependencies can cause compatibility issues, resulting in unexpected behavior and errors. Deprecated packages can be more prone to bugs and security vulnerabilities, and may not have the latest features available. They can be more difficult to debug, and support assistance might be lacking.
So keep your dependencies up-to-date for a performant, reliable, and secure application.
Major Vs. Minor Version Changes
When making changes to an application, you need to understand the difference between major and minor changes.
Major version updates usually contain backward-incompatible changes to a library (e.g., function/class name changes, function/class deletions, changes in function parameters or return types, etc.).
When you update to a major version of a library in your project, it's likely the code using that library won't work well with the new library.
Minor changes, on the other hand, are backward-compatible and won't break your code.
As for upgrading packages, most package publishers use Semantic Versioning (SemVer for short) to identify whether an update introduces breaking changes. The version is formatted as MAJOR.MINOR.PATCH
:
MAJOR
version changes introduce incompatible API changesMINOR
version changes add functionalities that are backward-compatiblePATCH
version changes make backward-compatible bug fixes
It is a good practice to write code tests that might notify you of any issues after a package update.
Making Sure Your Dependencies Stay Up-To-Date
To ensure that your dependencies stay up-to-date, there are several steps you can take:
- Create a process to regularly check for updates and ensure that it is followed.
- Use version control to keep track of the versions of packages and dependencies being used. Version control allows you to easily test different versions of dependencies in your project and it also enables you to take your app back to a stable state, in cases where a problematic dependency or update was introduced. A package manager such as
npm
oryarn
can automate the process of updating packages and dependencies. - Consider using a vulnerability scanner such as Snyk to identify potential security risks.
Now let's turn our attention to another potential pitfall in Node: memory leaks.
Memory Leaks in Node.js
Memory leaks can be caused by objects that are no longer being used but still exist in memory.
Memory leaks occur when an application continues to allocate more and more memory over time, but fails to release it when it is finished with it. This can cause an application to become sluggish and eventually crash.
Common causes of memory leaks include:
- Not properly disposing of objects.
- Forgetting to release references to objects.
- Improperly managing callbacks.
How to Manage Memory Allocation
To grasp memory leaks, you should familiarize yourself with memory management in Node.js. This entails understanding how the V8 Engine (the JavaScript engine used by Node.js) manages memory.
Memory allocation is a broad topic. Our article Avoiding Memory Leaks in Node.js: Best Practices for Performance will give you some initial pointers about how to handle memory leaks.
Monitoring Memory Leaks with AppSignal
AppSignal can be used to monitor memory leaks in Node.js applications. It’s designed to help developers identify and fix memory leaks quickly and easily.
AppSignal provides detailed information about memory usage, including total memory used, the amount of memory allocated, and the most frequent allocations. It also pinpoints the code and call stack causing the memory leak.
Here is how a heap size graph looks in AppSignal:
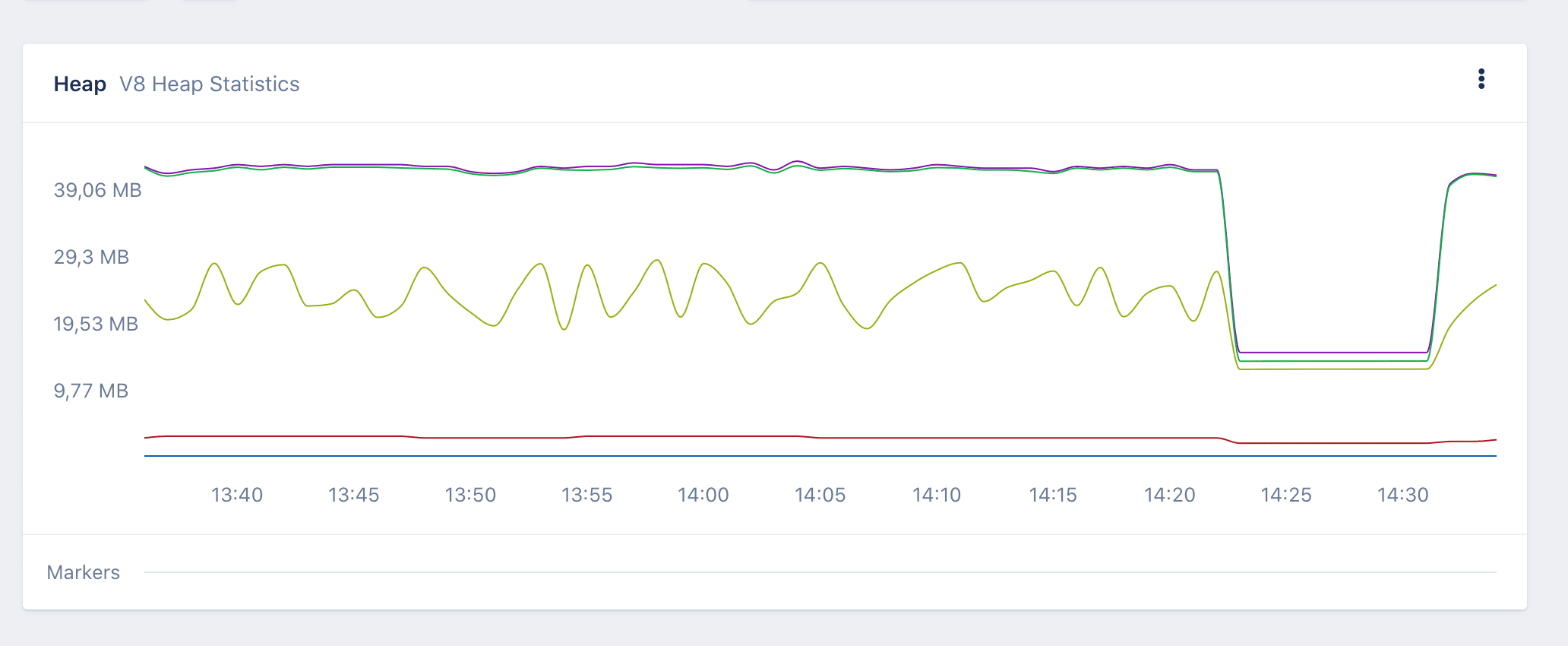
You can set alerts when specific values trigger set thresholds.
The next possible pitfall we'll look at is improper error handling.
Poor Error Handling in Node.js
Error handling is an important part of any program, and Node.js is no exception. Poor error handling can cause your application to crash. You must properly handle errors to prevent them from propagating up the stack.
Handle errors as close to their source as possible. Failing to do so can cause memory leaks, stability issues, and bugs within your application. Log errors to help identify and debug issues quickly and easily.
Best Practices for Error Handling
It is a good practice to handle errors using the error-first callback pattern. This pattern involves passing an error object as the first argument to a callback function and checking for the existence of an error, before proceeding with any further logic.
const errorFirstCallback = (err, data) => { if (err) return console.log(err); console.log("It works!"); }; wafel.addTopping(topping, errorFirstCallback);
Don't swallow errors — that means, don't use try-catch blocks. Instead, let an error bubble up and be handled by the caller.
Having a robust and comprehensive error-handling strategy in place will ensure that your application stays safe and can handle any unexpected errors. See Node.js Error Handling: Tips and Tricks for a summary of what to consider.
Here are some things to keep in mind:
- Log errors and their stack traces to help track down the cause of an issue.
- Define custom error classes to differentiate between different types of errors.
- Wrap callback functions with a try-catch block to handle errors properly.
- Return early from functions to avoid deep nesting.
- Avoid throwing errors from within callbacks.
- Use
Promise.catch()
to catch errors with Promises. - Handle errors that are thrown from asynchronous code.
- Handle errors gracefully, and provide useful feedback to the user.
Tracking Errors with AppSignal
Tools such as AppSignal can help centralize error handling in your application. It can capture and log unhandled errors, as well as provide helpful debugging information.
AppSignal provides detailed error reports that include stack trace, metadata, and diagnostic information, like the environment in which an error occurs and the request that caused it.
Here's an example showing the details of an error in AppSignal:
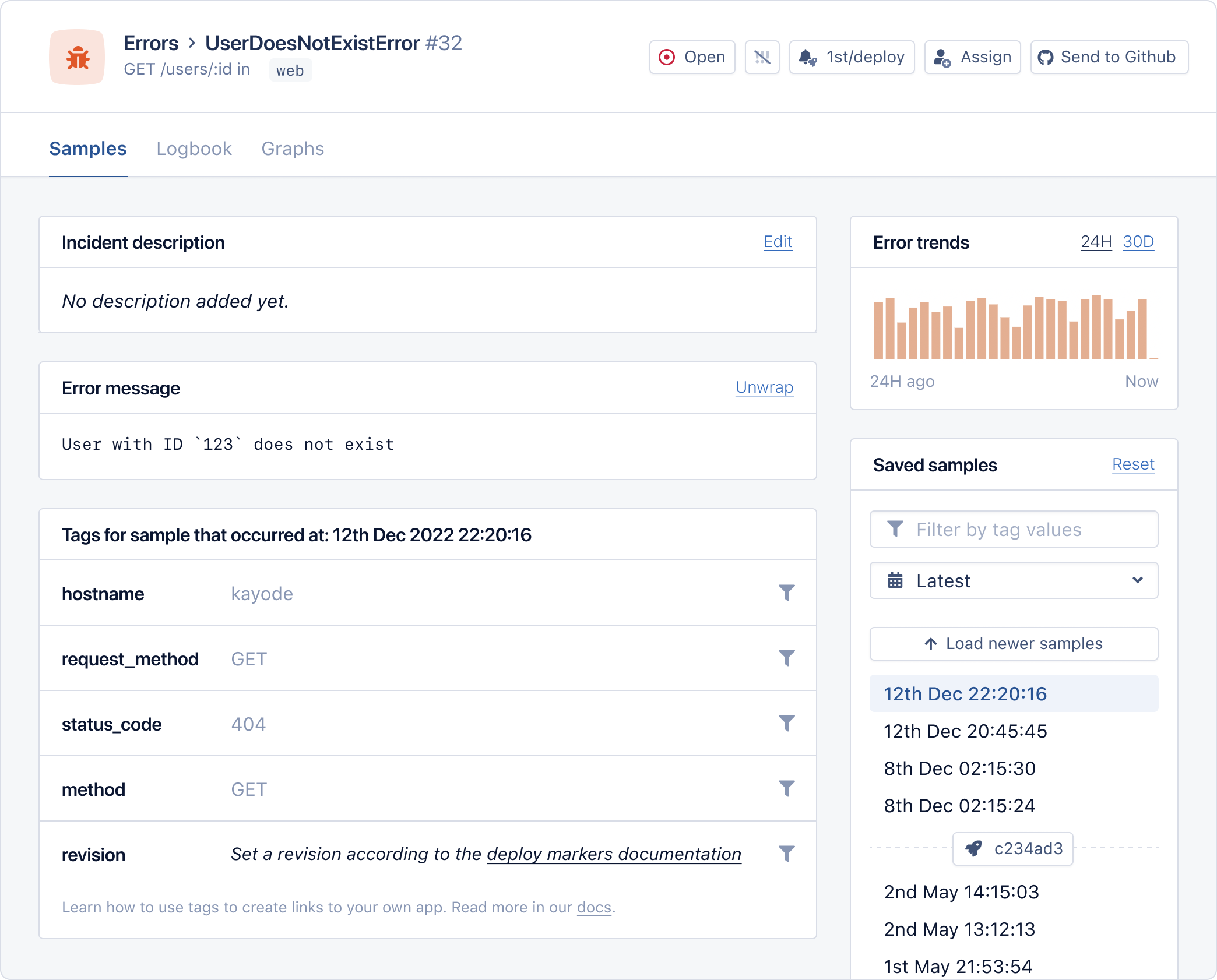
Now let's move on to another potential problem you might fall prey to: writing overly complex or unreadable code.
Overcomplicated and Unreadable Code
Badly written and unreadable code can cause a variety of issues, ranging from performance issues to security vulnerabilities. It can be difficult to debug and maintain, and leads to errors and unexpected behavior.
When writing code, it is important you keep it clean and organized. This means breaking code into modules, using descriptive variable and function names, and avoiding deep nesting. This will make the code easier to debug. Additionally, using comments is a great way to make code more understandable.
Following Don’t Repeat Yourself (DRY)/Duplication is Evil (DIE), Keep it Simple, Stupid (KISS), and SOLID principles can ensure better code quality.
Development tools such as linters identify badly written code and inconsistencies and suggest improvements to improve code readability.
Regular code reviews also ensure that code is written properly and is easy to read.
Here's an example of code that's badly-written and well-written:
// ❌ Bad code function doSomething(a, b, c) { let x = a + b; let y = x * c; let z = y / 2; return z; } // ✅ Good code function calculateResult(num1, num2, num3) { let sum = num1 + num2; let product = sum * num3; let halfProduct = product / 2; return halfProduct; } // The first code block is difficult to read due to the lack of descriptive variable names.
The next thing to consider is your security practices.
Security Practices and Measures for Your Node.js Application
Neglecting to address security issues can lead to data breaches, data loss, and other serious consequences for your Node.js application. By taking the necessary precautions, your application stays secure. Here are a few practices to follow.
Avoid Injection Attacks
Injection attacks are when an attacker injects malicious code into an application through user input.
Prevent this by using prepared statements or parameterized queries and properly sanitizing user input.
Prevent Insecure Secrets
Secrets like API keys or database credentials stored in plain text or in the codebase are considered insecure secrets.
You can avoid this by using environment variables or a secrets management solution.
Use HTTPS and SSL/TLS
Using HTTPS and SSL/TLS is essential for encrypting data sent between a server and a client. This protects sensitive data such as passwords and credit card numbers.
Utilize Security Headers
Security headers are HTTP headers that help secure an application. These headers can provide additional security measures, such as prohibiting content from being embedded on other sites, preventing the browser from executing malicious code, and preventing clickjacking attacks.
Set Up Access Control
Access control ensures that only authorized users can access certain resources. Setting up proper access control will ensure that unauthorized users cannot access sensitive data.
Make Use of Encryption
Encrypting data is an important security measure. Encryption ensures that data is not readable by anyone other than the intended recipient.
Perform Security Audits
Perform regular security audits, both manually and with automated tools, to identify any potential security issues.
We'll now focus on another problem you might run into: blocking code.
Blocking Code
Blocking code blocks the main thread from executing other tasks while it is running. This can cause performance issues, as an application cannot respond to requests or perform other tasks until the blocking code has finished running.
The main causes of blocking code include long-running loops and synchronous functions. Nested loops can take a long time to run, so an application becomes unresponsive as the main thread is occupied in executing the loop. Synchronous functions cause a block as an application will wait for a function to finish executing before moving on to the next task.
To avoid blocking code, use asynchronous functions whenever possible. Avoid using highly nested loops by breaking them up into separate loops using recursion or another better-performing algorithm. Additionally, consider using a task queue to separate long-running tasks from the main thread.
// ❌ Blocking, synchronous code const fs = require("fs"); const data = fs.readFileSync("/wafel.txt"); // Blocks here until the file is read // ✅ Non-blocking, asynchronous code const fs = require("fs"); fs.readFile("/wafel.txt", (err, data) => { if (err) throw err; });
To better understand asynchronous code, refer to How to Handle Async Code in JavaScript.
Next up is an issue you're likely familiar with: callback hell.
Callback Hell
Callback hell describes a program with an excessive number of nested callbacks, resulting in code that is difficult to read and maintain. This can be caused by poorly structured code or too many asynchronous functions.
Callbacks are used to handle asynchronous operations, and they can be nested to allow for complex operations. However, excessive nesting of callbacks can lead to confusing and unreadable code. It can also lead to errors and unexpected behavior, making debugging and maintaining such code difficult.
To avoid callback hell, structure code properly and use other techniques such as Promises, async/await, and generators.
// ❌ Callback hell fs.readFile("wafel1.txt", (err, data1) => { if (err) { console.error(err); return; } fs.readFile("wafel2.txt", (err, data2) => { if (err) { console.error(err); return; } fs.readFile("wafel3.txt", (err, data3) => { if (err) { console.error(err); return; } fs.readFile("wafel4.txt", (err, data4) => { if (err) { console.error(err); return; } // Do something with data1, data2, data3, and data4 }); }); }); }); async.waterfall( [ (callback) => fs.readFile("wafel1.txt", callback), (data1, callback1) => fs.readFile("wafel2.txt", callback1), (data2, callback2) => fs.readFile("wafel3.txt", callback2), (data3, callback3) => fs.readFile("wafel4.txt", callback3), ], (err, data4) => { if (err) { console.error(err); return; } // Do something with the data } );
Promises and callback hell are also covered in How to Handle Async Code in JavaScript. For a more detailed approach to callback hell, refer to callbackhell.com.
Finally, we'll look at another common problem: overusing global variables.
Overuse of Global Variables
Global variables are available to an entire program. They are useful for storing data that may be needed in multiple places throughout the program. However, overusing global variables can lead to issues such as increased complexity and security risks.
Using global variables can make code difficult to debug and maintain, as they can be accessed from anywhere in a program. Changes to a global variable in one part of the program can have unexpected consequences elsewhere.
Overusing global variables can introduce vulnerabilities in a program. This is because global variables usually disconnect a source of data from its usage and make it difficult to track all the places in your code where the variable can be modified. So a value that comes from an unsafe source (e.g., user input) could be used in the same way as a value from a safer source (e.g., a database or calculation), without the added safety checks that should be used on such values. This leaves your program vulnerable to malicious actors.
Limit your use of global variables to only when they are absolutely necessary. When possible, variables should be passed as arguments to functions instead of being stored as global variables.
Wrapping Up
In this article, we discussed several Node.js pitfalls that you should avoid, including:
- having outdated dependencies
- memory leaks
- improper error handling
- overcomplicated code
- neglecting security practices
- blocking code
- callback hell
- the overuse of global variables
To maintain a secure and reliable application, ensure your code is readable and maintainable, follow good coding practices, and use asynchronous functions and security measures.
Happy coding!
P.S. If you liked this post, subscribe to our JavaScript Sorcery list for a monthly deep dive into more magical JavaScript tips and tricks.
P.P.S. If you need an APM for your Node.js app, go and check out the AppSignal APM for Node.js.