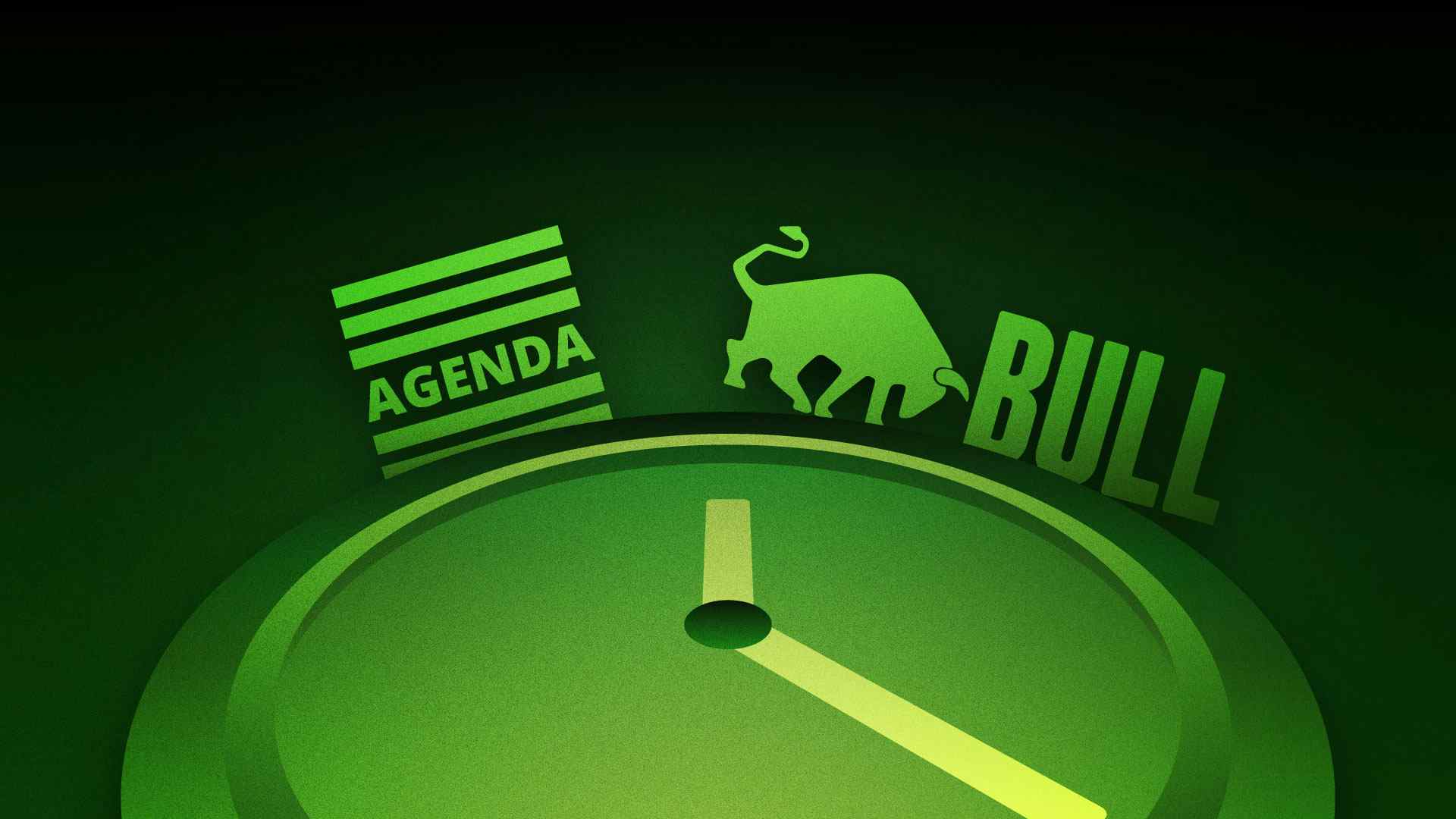
Whether you're familiar with job schedulers or new to the concept, two popular libraries in the Node.js ecosystem stand out: Bull and Agenda.
These libraries provide unique features and advantages when it comes to efficient job scheduling. Let's explore the ins and outs of Bull and Agenda! ๐
Scheduling Jobs in Node.js: Conducting An Orchestra
A job scheduler can be likened to an orchestra conductor in a Node.js application. Just as a conductor leads and coordinates musicians to produce a harmonious symphony, a job scheduler orchestrates the execution of tasks in your application.
Imagine your application as a grand orchestra, with each task representing a skilled musician. Without a conductor, each musician might play their part at their own pace, resulting in a disjointed and chaotic performance. Similarly, without a job scheduler, tasks in your application may run independently, leading to resource conflicts, delays, and suboptimal performance.
Just as a conductor adapts to unforeseen circumstances during a live performance, a job scheduler handles exceptions and failures in your application. It gracefully manages errors, reschedules failed tasks, and ensures that the show goes on smoothly, maintaining the reliability and resilience of your application.
Now that we've summarised the role of a job scheduler, let's turn our attention to what Bull can do.
Bull for Node.js
Bull claims to be the fastest, most reliable Redis-based queue for Node.js. It is known for its high performance, scalability, and robustness. With Bull, you can create queues to manage and process jobs asynchronously, making it ideal for handling time-consuming or resource-intensive tasks.
Benefits of Bull as a Job Scheduler
Using Bull as a job scheduler brings several benefits to your Node.js application:
- High performance and scalability: Bull leverages efficient algorithms and data structures to process jobs quickly. It is designed to handle high workloads and scales horizontally.
- Reliable job processing and job queues: Bull ensures reliable job processing by providing features such as job persistence, automatic retries, error handling, and priority queues.
- Advanced job scheduling options: You can schedule jobs to run at specific times, set recurring jobs using cron-like expressions, or define intervals between job executions.
- Monitoring and insights: Bull provides a built-in monitoring dashboard to track job progress, view statistics, and monitor performance metrics. This feature allows you to gain insights into the job processing pipeline, identify bottlenecks, and optimize your application's performance.
- Integration and compatibility: Bull integrates well with popular frameworks like Express or Nest.js and can easily integrate with different data stores, message brokers, and external services.
- Extensive ecosystem and community support: Bull is actively maintained and regularly updated, ensuring compatibility with the latest versions of Node.js. The community provides helpful resources, documentation, and plugins to extend Bull's functionality and address various use cases.
Take note, however, of the following information from the Bull documentation:
Bull is currently in maintenance mode, we are only fixing bugs. For new features check BullMQ, a modern rewritten implementation in Typescript. You are still very welcome to use Bull if it suits your needs, which is a safe, battle-tested library.
Real-world Use Cases Where Bull Shines
Bull excels in real-world use cases where efficient job scheduling and task management are critical. In distributed systems or microservice architectures, Bull particularly shines as a job scheduler for coordinating and synchronizing tasks across multiple services or nodes. Here's a code example:
// Service A - Producer const Queue = require("bull"); // Create a Bull queue const queue = new Queue("taskQueue", { redis: { host: "localhost", port: 6379, }, }); // Producer service adds jobs to the queue async function addToQueue(data) { await queue.add(data); console.log("Job added to the queue:", data); } // Service B - Consumer // Create a Bull queue with the same name as the producer const queue = new Queue("taskQueue", { redis: { host: "localhost", port: 6379, }, }); // Consumer service processes jobs from the queue queue.process(async (job) => { console.log("Processing job:", job.data); // Perform the necessary actions for the job // ... // Mark the job as completed return Promise.resolve(); }); // Start producing jobs addToQueue({ data: "Task 1" }); addToQueue({ data: "Task 2" }); addToQueue({ data: "Task 3" });
In this example, we have two services, Service A (Producer) and Service B (Consumer). They both share a Bull queue named taskQueue
. Service A produces jobs by adding data to the queue using the addToQueue
function. Service B consumes these jobs and processes them using the queue.process
method.
For instance, when addToQueue({ data: 'Task 1' })
is called, it adds a job to the Bull queue named taskQueue
. The job contains data { data: 'Task 1' }
representing the specific task or job to be processed.
The queue.add(data)
method adds the job to the queue, and Bull takes care of persisting the job details in the underlying Redis database. The job is then available for consumption by any connected consumer service processing jobs from the same queue.
When queue.process
is called in the consumer service, it sets up a worker that listens to the queue and starts processing jobs as they become available. In this case, the worker will process the job containing { data: 'Task 1' }
once it reaches the front of the queue.
The job processing involves invoking the function provided to queue.process
. In the code example, the function logs the job data (console.log('Processing job:', job.data)
) and performs any necessary actions specific to the job.
By calling addToQueue
with different data for each task, you can add multiple jobs to the queue. The consumer service will process them one by one based on the order in which they were added.
Other use cases where Bull shines include:
- Background processing and task offloading
- Task scheduling and automation
- Job queues and workload balancing
- Delayed and retry mechanisms
But, as the saying goes: "Every rose has its thorn". Bull, while an exciting choice of job scheduler, also has some noteworthy drawbacks.
Potential Drawbacks of Bull
- Dependency on Redis: Bull relies on Redis as its underlying data store, requiring the setup and maintenance of a Redis server. This can introduce added complexity and infrastructure demands if you're not already using Redis in your application.
- Steep learning curve: Bull's wide range of features and advanced options may require developers new to job scheduling and asynchronous task management to invest time in learning and experimenting to utilize its capabilities effectively.
- Memory consumption: Bull's memory usage can increase with large job queues, necessitating careful monitoring and optimization to stay within acceptable limits, particularly in memory-constrained or high-throughput environments.
- Persistence options: Bull's reliance on Redis for persistence may not align with your preferred data store, potentially necessitating custom solutions or alternative job scheduler libraries to integrate with a different database system.
- Overkill for simple use cases: If your application has straightforward scheduling requirements or doesn't require advanced job processing features, integrating Bull may introduce unnecessary complexity. In such cases, a simpler job scheduler or task queue solution might be more suitable.
This brings us to Bullโs alternative โ Agenda.
Agenda for Node.js
Agenda is a lightweight job scheduling library for Node.js, built on top of MongoDB. It focuses on simplicity and ease of use, making it an excellent choice for applications that require basic job scheduling capabilities without the need for complex features.
Benefits of Agenda as a Job Scheduler
Here are a few notable benefits of using Agenda:
- Simple and lightweight: Agenda is designed to be lightweight and easy to use, making it a great choice for applications with simpler job scheduling requirements.
- Persistence options: Unlike Bull, Agenda supports multiple databases, including MongoDB as the default choice. This gives you the flexibility to use your preferred database system for storing job data.
- Event-driven architecture: Agenda's event-driven architecture enables seamless integration and efficient communication between application components through custom event-triggered job execution.
- Error handling and job recovery: Agenda includes built-in error handling, job recovery, retries, and concurrency control, ensuring effective management of job execution errors and recovery from failures in your application.
- Job scheduling flexibility: Agenda provides comprehensive scheduling capabilities, human-readable syntax, and complex recurring schedules for executing tasks at regular intervals or specific times.
- Active community and maintenance: Agenda has an active community and ongoing maintenance, ensuring regular updates, bug fixes, ample resources, documentation, and community support for any issues or assistance you may need.
Examples Where Agenda Is a Good Fit
Agenda is well-suited for a range of scenarios, including recurring tasks, cron jobs, event-driven workflows, and lightweight use cases that demand a straightforward API and a minimalistic solution for job scheduling in your application.
The following piece of code uses Agenda to execute tasks based on complex time patterns (using cron syntax):
const Agenda = require("agenda"); const agenda = new Agenda(); // Define a job to be executed based on cron schedule agenda.define("sendEmail", async (job) => { const { to, subject, body } = job.attrs.data; // Send email logic here console.log(`Sending email to ${to} with subject: ${subject}`); }); // Define the cron schedule for the job agenda.every("0 8 * * *", "sendEmail", { to: "example@example.com", subject: "Daily Report", body: "Hello, this is your daily report.", }); // Start the agenda instance and execute jobs (async () => { await agenda.start(); console.log("Agenda started"); // Optionally, you can define additional jobs and schedules here // Gracefully shut down the agenda instance await agenda.stop(); })();
In this example, we define an Agenda instance and then define a job named sendEmail
. We specify the job's logic inside the async function. The every
method is used to schedule the job with the cron expression 0 8 * * *
, which means that the job will run every day at 8:00 AM. The job data includes the email recipient, subject, and body.
When the agenda starts, it will execute the job according to the defined schedule. You can add more jobs and schedules as needed within the async block. Finally, the optional await agenda.stop();
is called to gracefully stop the Agenda instance when the application is finished.
Limitations of Agenda
While Agenda offers several advantages, it also comes with some trade-offs to consider:
- Lack of advanced features: If your application requires complex job orchestration, prioritization, or extensive queue management, you may find Agenda's feature set to be less comprehensive than Bull's.
- Single process execution: By default, Agenda operates within a single process, which simplifies deployment and setup but may not scale as effectively as Bull in terms of handling high job concurrency and distributed processing.
- Less active community: While Agenda has an active community, it may have a smaller user base compared to other popular alternatives like Bull, potentially resulting in fewer available resources, documentation, and community support.
- Learning curve for complex scenarios: Agenda is user-friendly for simpler use cases, but can have a steeper learning curve for complex job scheduling needs. If your application requires intricate workflows, advanced scheduling patterns, or sophisticated error handling, a deeper understanding of Agenda's capabilities may be necessary.
Feature Comparison between Bull and Agenda for Node.js
Here's a concise table comparing the features of each library to help you choose the optimal job scheduler for your Node.js projects.
Let's dive into the key similarities and differences between Bull and Agenda to make an informed choice! ๐
Feature | ๐ Bull | ๐๏ธ Agenda |
---|---|---|
Job Persistence | Redis | MongoDB (with support for other databases) |
Scalability | Suitable for high job concurrency | Single process, may not scale as effectively |
Learning Curve | Moderate | Relatively easy for simpler use cases |
Advanced Features | Extensive options for job management | Limited compared to more feature-rich schedulers |
Persistence Flexibility | Tightly integrated with Redis | Supports multiple databases, including MongoDB |
Event-Driven Architecture | Not emphasized | Embraces event-driven workflows |
Error Handling | Customizable error-handling mechanisms | Built-in error handling and job recovery options |
Community Support | Widely adopted, active community | Active community but with a smaller user base |
Scheduling Flexibility | Versatile scheduling options | Rich set of scheduling features, including cron |
Resource Consumption | Potentially higher memory consumption | Lightweight and optimized for lower resource usage |
Integration | Works well with existing Redis setups | Flexible integration with various tech stacks |
Use Case Suitability | Complex job orchestration and queueing | Simpler use cases and straightforward scheduling |
Ultimately, the choice between Bull and Agenda boils down to your project's specific needs and complexity. Whether you prioritize advanced features, scalability, simplicity, or specific integration requirements, use the insights gained from this comparison to make an informed decision that aligns with your development goals.
Remember, your choice of job scheduler plays a vital role in efficient task management. Choose wisely and enjoy seamless job scheduling in your Node.js applications.
Wrapping Up
In this post, we explored Bull and Agenda as job scheduler libraries for Node.js applications.
We learned about the benefits of Bull, such as reliable job persistence and extensive features, and its real-world use cases. Additionally, we discovered Agenda's advantages, including its support for multiple databases, event-driven architecture, and rich scheduling options.
By comparing their features, potential drawbacks, and use case suitability, we can now make informed decisions when choosing between Bull and Agenda for our specific application requirements.
Happy job scheduling!
P.S. If you liked this post, subscribe to our JavaScript Sorcery list for a monthly deep dive into more magical JavaScript tips and tricks.
P.P.S. If you need an APM for your Node.js app, go and check out the AppSignal APM for Node.js.